
如何用C语言实现gDialog对话框?
在C语言中使用gdialog
工具,可以通过调用系统命令来实现,以下是一个详细的示例,展示如何在C语言中使用gdialog
来创建不同类型的对话框,并进行交互操作。

使用gdialog创建消息框、输入框和菜单框的示例
1. 创建消息框
我们来看如何创建一个消息框,用于显示一条信息并等待用户点击“OK”按钮。
#include <stdio.h> #include <stdlib.h> int main() { char command[256]; // 创建消息框,内容为 "Hello, World!" snprintf(command, sizeof(command), "gdialog title \"Message Box\" msgbox \"Hello, World!\" 0 0"); system(command); return 0; }
这个程序会生成一个带有标题“Message Box”的消息框,显示“Hello, World!”的信息,并在用户点击“OK”后关闭。
2. 创建输入框
我们创建一个输入框,让用户输入一些文本,并将输入的内容保存到文件中。
#include <stdio.h> #include <stdlib.h> int main() { char command[256]; FILE *fp; char input[1024]; // 创建输入框,提示用户输入名字 snprintf(command, sizeof(command), "gdialog title \"Input Box\" inputbox \"Enter your name:\" 0 0 2> > input.txt"); system(command); // 读取用户输入的内容 fp = fopen("input.txt", "r"); if (fp == NULL) { perror("Error opening file"); return EXIT_FAILURE; } fgets(input, sizeof(input), fp); fclose(fp); // 打印用户输入的内容 printf("You entered: %s ", input); return 0; }
这个程序会生成一个带有标题“Input Box”的输入框,提示用户输入名字,并将输入的内容保存到input.txt
文件中,然后读取文件内容并打印出来。
3. 创建菜单框
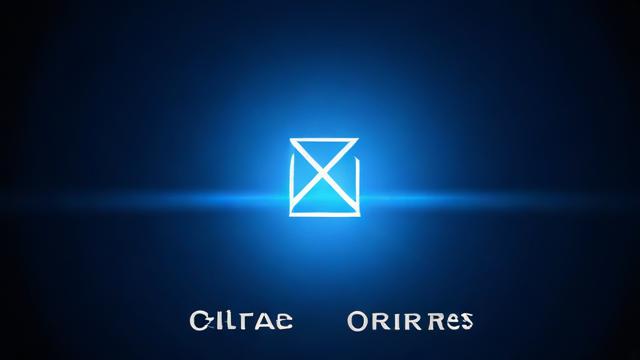
我们创建一个菜单框,让用户选择不同的选项。
#include <stdio.h> #include <stdlib.h> int main() { char command[256]; int choice; // 创建菜单框,提供两个选项:Calculate my GPA 和 Quit snprintf(command, sizeof(command), "gdialog title \"GPA calculator\" menu \"Menu\" 9 18 2 1 \"Calculate my GPA\" 2 \"Quit\" 2 > choice.txt"); system(command); // 读取用户的选择 FILE *fp = fopen("choice.txt", "r"); if (fp == NULL) { perror("Error opening file"); return EXIT_FAILURE; } fscanf(fp, "%d", &choice); fclose(fp); // 根据用户的选择执行相应的操作 if (choice == 1) { printf("You chose to calculate your GPA. "); // 这里可以添加计算GPA的逻辑 } else { printf("You chose to quit. "); } return 0; }
这个程序会生成一个带有标题“GPA calculator”的菜单框,提供两个选项:“Calculate my GPA”和“Quit”,并将用户的选择保存到choice.txt
文件中,然后读取文件内容并根据用户的选择执行相应的操作。
是使用C语言结合gdialog
工具创建消息框、输入框和菜单框的基本示例,通过这些示例,你可以看到如何使用系统命令来调用gdialog
并处理用户输入和选择,以下是一个简单的表格归纳:
功能 | 命令示例 | 描述 |
消息框 | gdialog title "Message Box" msgbox "Hello, World!" 0 0 | 显示消息并等待用户点击“OK”按钮 |
输入框 | gdialog title "Input Box" inputbox "Enter your name:" 0 0 2> > > input.txt | 提示用户输入文本并保存到文件 |
菜单框 | gdialog title "GPA calculator" menu "Menu" 9 18 2 1 "Calculate my GPA" 2 "Quit" 2 > choice.txt | 提供菜单选项并保存用户选择 |
这些示例展示了如何在C语言中利用gdialog
进行简单的图形界面交互,你可以根据需要进一步扩展这些示例,实现更复杂的功能。
文章版权及转载声明
作者:豆面本文地址:https://www.jerry.net.cn/articals/11658.html发布于 2025-01-06 00:58:48
文章转载或复制请以超链接形式并注明出处杰瑞科技发展有限公司